Eine Einfache „Hallo-World“ Ausgabe ist doch etwas langweilig, der motivierte Einsteiger kann sich auch ein einfaches Programm zumuten, mit dem man interagieren kann. Ich zeige zwei kleine Beispiele für ein einfaches Andoid-Progamm.
Hello Progress Dialog
Mit einem Button öffnet sich ein kleiner Hinweis-Dialog, das uns mitteilt dass Android beschäftigt ist. Vor allem bei Online-Anwendungen, wenn die Verbindung schlecht ist, dauert es eine Weile bis Informationen aus dem Internet vollständige geladen sind. Solch ein Hinweis beruhigt den Benutzer, indem es ihm klar macht, dass im Hintergrund noch etwas passiert.
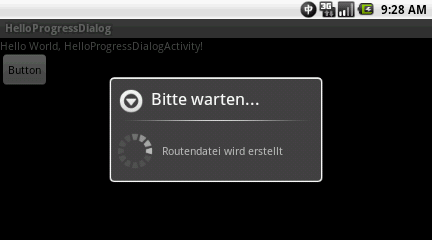
package tk.pixilab.android;
import android.app.Activity;
import android.app.ProgressDialog;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
public class HelloProgressDialogActivity extends Activity {
private ProgressDialog verlauf;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Button sfDateiAnlegen
= (Button) findViewById
(R.
id.
sf_dateiAnlegen); sfDateiAnlegen.setOnClickListener(new OnClickListener() {
@Override
public void onClick
(View v
) { verlauf = ProgressDialog.show(HelloProgressDialogActivity.this,
"Bitte warten...",
"Routendatei wird erstellt");
public void run() {
speichereTestroute();
verlauf.dismiss();
}
private void speichereTestroute() {
try {
sleep(10000);
};
}.start();
}
});
}
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<Button android:text="Button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/sf_dateiAnlegen"></Button>
</LinearLayout>
Hello Popup
Auch auf kleinen Smartphones gibt es Popups, so ähnlich wie das Hinweis-Fenster das man oben gesehen hat. Probiert es doch mal aus.
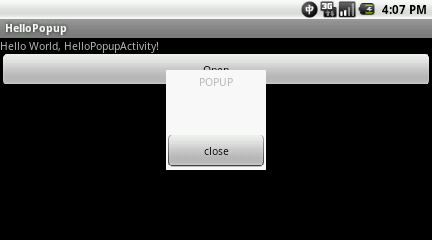
package tk.pixilab.android;
import android.app.Activity;
import android.content.Context;
import android.os.Bundle;
import android.view.Gravity;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.Button;
import android.widget.PopupWindow;
import android.widget.Toast;
public class HelloPopupActivity extends Activity {
private Button openPopup, closePopup
;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
myPopup();
}
private void myPopup() {
final LayoutInflater inflater
= (LayoutInflater
) HelloPopupActivity.
this.
getSystemService(Context.
LAYOUT_INFLATER_SERVICE); final View popupView
= inflater.
inflate(R.
layout.
popup,
null,
false); final PopupWindow pw = new PopupWindow(popupView, 100, 100, true);
openPopup
= (Button) findViewById
(R.
id.
openPopup); openPopup.setOnClickListener(
new View.
OnClickListener() { @Override
public void onClick
(View v
) { pw.showAtLocation(findViewById(R.id.main), Gravity.CENTER, 0,0);
Toast.makeText(getApplicationContext(), "popup opened", Toast.LENGTH_LONG).show();
}
}
);
closePopup
= (Button) popupView.
findViewById(R.
id.
closePopup); closePopup.setOnClickListener(
new View.
OnClickListener() { @Override
public void onClick
(View v
) { Toast.makeText(getApplicationContext(), "popup closed", Toast.LENGTH_LONG).show();
pw.dismiss();
}
}
);
}
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:id="@+id/main"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<Button
android:layout_width="fill_parent"
android:id="@+id/openPopup" android:text="Open" android:layout_height="wrap_content"/>
</LinearLayout>
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:background="#FFFFFF" android:layout_width="200dip" android:layout_height="300dip">
<Button android:text="close" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:layout_width="fill_parent" android:id="@+id/closePopup"></Button>
<TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/textView1" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" android:text="POPUP" android:padding="5dip"></TextView>
</RelativeLayout>